Table of Contents
ToggleIntroduction
In the realm of backend development, where numerous frameworks have come and gone, one rising star shines brightly: NestJS. With its blend of remarkable features, unparalleled scalability, and exquisite developer experience, NestJS is undeniably the next big thing that is redefining the landscape of server-side development.
A Framework That Speaks TypeScript
NestJS, built on the rock-solid foundation of Node.js, is a versatile and robust framework that leverages TypeScript to enhance code quality and maintainability. By embracing TypeScript’s static typing, NestJS enables developers to catch errors early, promotes modular code organization, and facilitates easier refactoring. Let’s take a look at a simple example of a NestJS controller:
import { Controller, Get } from '@nestjs/common'; @Controller('hello') export class HelloController { @Get() getHello(): string { return 'Hello, World!'; } }
In this snippet, we define a basic controller using NestJS decorators. The @Controller decorator marks the class as a controller, and the @Get decorator specifies that the get hello () method should handle HTTP GET requests to the ‘/hello’ route. With just a few lines of code, we have a functional API endpoint.
Decorators: The Secret Sauce
One of the standout features of NestJS is its innovative use of decorators. These magical annotations elegantly transform plain classes into powerful modules, controllers, or services, simplifying the development process. With decorators, Nest JS gracefully handles dependency injection, routing, validation, and much more, resulting in clean and maintainable code. Consider this example:
import { Injectable } from '@nestjs/common'; @Injectable() export class GreetingService { getGreeting(name: string): string { return `Hello, ${name}!`; } }
Here, the @Injectable() decorator marks the class as a service that can be injected into other components. The GreetingService can now be easily used by other classes or controllers without worrying about managing dependencies manually.
Scalability Redefined
Nest JS’s modular architecture and layered approach provide unparalleled scalability. By implementing the principle of separation of concerns, it allows developers to create loosely coupled modules that can be independently developed and tested. These modules seamlessly integrate, providing a solid foundation for microservices and facilitating effortless horizontal scaling. NestJS ensures your application can gracefully handle an influx of users, like a well-organized tea party that accommodates unexpected guests with elegance.
Unleashing the Power of WebSockets
Real-time communication is the flavor of modern web applications, and NestJS has a knack for handling it with grace. With built-in support for WebSockets, NestJS enables bidirectional communication, transforming your applications into live, interactive experiences. Consider this example of a WebSocket gateway:
import { WebSocketGateway, WebSocketServer } from '@nestjs/WebSockets; import { Server } from 'socket.io'; @WebSocketGateway() export class ChatGateway { @WebSocketServer() server: Server; handle connection() { // Handle new WebSocket connection } handleDisconnect() { // Handle WebSocket disconnection } }
In this snippet, we define a WebSocket gateway using the @WebSocketGateway() decorator. The handleConnection() and handleDisconnect() methods are called when a client connects or disconnects from the WebSocket server. Nest JS simplifies the implementation of real-time features, like chatting or live updates, with ease.
A Community That Brews Brilliance
Behind the success of any framework lies a vibrant and passionate community, and Nest JS is no exception. The community actively contributes to an extensive ecosystem of plugins, 0 libraries, and tools, enriching the framework’s capabilities. Developers can tap into this wealth of resources, leveraging battle-tested solutions and benefiting from the collective wisdom of the community.
Advantages Over Other Frameworks
-
- TypeScript Love: Nest JS’s strong integration with TypeScript ensures a better developer experience by catching errors at compile time, promoting code consistency, and providing excellent tooling support. This advantage gives NestJS an edge over frameworks that rely solely on JavaScript.
- Scalable and Modular Architecture: Nest JS’s modular architecture, inspired by Angular, enables developers to build scalable and maintainable applications. The use of modules, controllers, and services promotes code organization and the separation of concerns, making it easier to manage complex applications and facilitating effortless horizontal scaling.
- Developer-Friendly Decorators: Nest JS’s innovative use of decorators simplifies common tasks such as routing, dependency injection, validation, and more. By leveraging decorators, developers can write clean and concise code, improving productivity and reducing boilerplate code compared to other frameworks.
- Out-of-the-Box WebSockets: NestJS provides built-in support for WebSockets, allowing developers to easily implement real-time communication in their applications. This feature eliminates the need for additional libraries or complex configurations, giving Nest JS an advantage over frameworks that lack native WebSocket support.
- Vibrant and Supportive Community: NestJS benefits from a thriving community of developers who actively contribute to its growth. The community provides support, shares knowledge, and develops a rich ecosystem of libraries and plugins, ensuring that developers have the resources they need to build robust applications.
- Seamless Integration with Existing Libraries: NestJS seamlessly integrates with various libraries and tools, allowing developers to leverage existing solutions and maintain compatibility with their preferred technology stack. This advantage makes Nest JS a flexible choice for developers working on projects with specific requirements or using specific libraries.
Latest Updates and Support
NestJS continues to evolve rapidly, with frequent updates and additions to its feature set. The community actively contributes to the framework’s development, ensuring that it stays relevant and up-to-date with the latest industry trends. Additionally, NestJS offers comprehensive documentation, tutorials, and a vast array of online resources to support developers in their journey.
Furthermore, NestJS provides out-of-the-box support for various technologies, including:
- Databases: Nest JS seamlessly integrates with popular databases like PostgreSQL, MySQL, MongoDB, and more. It offers modules and decorators that simplify database operations, allowing developers to focus on building robust applications.
- Authentication and Authorization: NestJS provides built-in support for authentication and authorization mechanisms, including OAuth, JWT, and Passport. It simplifies the implementation of secure user authentication and authorization workflows, ensuring the protection of sensitive data.
- Testing: NestJS places a strong emphasis on testability, providing developers with the tools and structures necessary for effective testing. The framework offers a robust testing module that facilitates unit testing, integration testing, and end-to-end testing. With built-in support for testing utilities like Jest, developers can easily write comprehensive tests for their Nest JS applications, ensuring the reliability and stability of the codebase.
Conclusion
NestJS, with its elegant and efficient approach to backend development, is setting new standards in the industry. Its seamless integration with TypeScript, modular architecture, and support for WebSockets make it a powerful framework for building scalable and maintainable applications. With a supportive community and advantages over other frameworks, NestJS is undoubtedly the next big thing in the world of server-side development. So, dive into NestJS and experience the elegance and efficiency it brings to your backend projects.
Authors
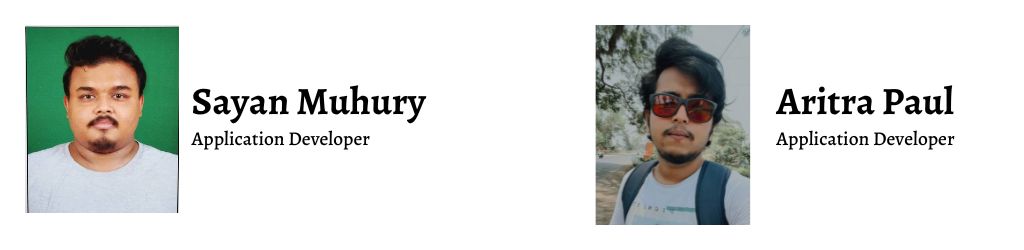