Unit testing is a mandatory part of software development done by the application developer. It actually ensures the functionality of an application, or more precisely, we can say that unit testing is a critical process to identify and resolve issues or bugs before the product is publicly released, i.e., it is done during the development phase of an application by the developer, which helps us guarantee the quality of our product. In one word, we can say unit testing is testing the smallest testable unit of an application.
There are many unit testing frameworks for Java Script, such as :
- Jest
- Mocha
- Jasmine
Table of Contents
ToggleFeatures Of Jest Framework
Jest is one of the most popular testing frameworks that was developed by Facebook. It is built on top of Jasmine, and it also provides additional features that make it easy to write and run tests. Other features provided by Jest are:
- test coverage reporting
- mocking
- snapshot testing
If we want to work with Jest, these are the steps that we need to follow for environment setup and to run the test cases:
- need to install node
- Install Jest as a dev dependency by using the following command:
npm install –save-dev jest
- Need to create a folder ‘test’ and under that need to create all test files with extensions .spec.js/.test.js
- In the package.json file, need to add
"scripts": {
"test": "jest”
}
- Run the tests by running the following command in your project directory: npm run test
With the help of the below example, we can demonstrate that:
add.js
function add(a, b) {
return a + b;
}
module.exports = { add };
Now, let’s write a Jest unit test for this function inside a directory, namely ‘test’:
test/add.spec.js
const { add } = require('../add');
describe('add function', () => {
it('should add two numbers', () => {
expect(add(100, 200)).toEqual(300);
});
it('adds 100 + 200 to equal 300', () => {
expect(add(100, 200)).toBe(300);
});
test('adds -100 + 200 to equal 100', () => {
expect(add(-100, 200)).toBe(100);
});
});
describe('add function', () => {
it('should add two numbers', () => {
const mockAdd = jest.fn(() => 100);
expect(mockAdd(100, 200)).toEqual(100);
expect(mockAdd).toHaveBeenCalledWith(100, 200);
});
});
Here, we are using Jest’s describe function to define the test suite and individual test case, respectively. Expect functions is using to test the output of the add function. Jest provides a wide range of matchers, including toEqual, toBe, toBeNull, etc. Mocking is another important jest feature. Mocking is the process of replacing a function with a fake implementation to test the behavior of a code unit.
Here, mocking is used to replace the add function with a custom implementation that always returns 100. We are then testing the behavior of the mock function by calling it with the arguments (100, 200) and using the toEqual matcher to check the output. Jest will run the tests and report whether they pass or fail by the below output:
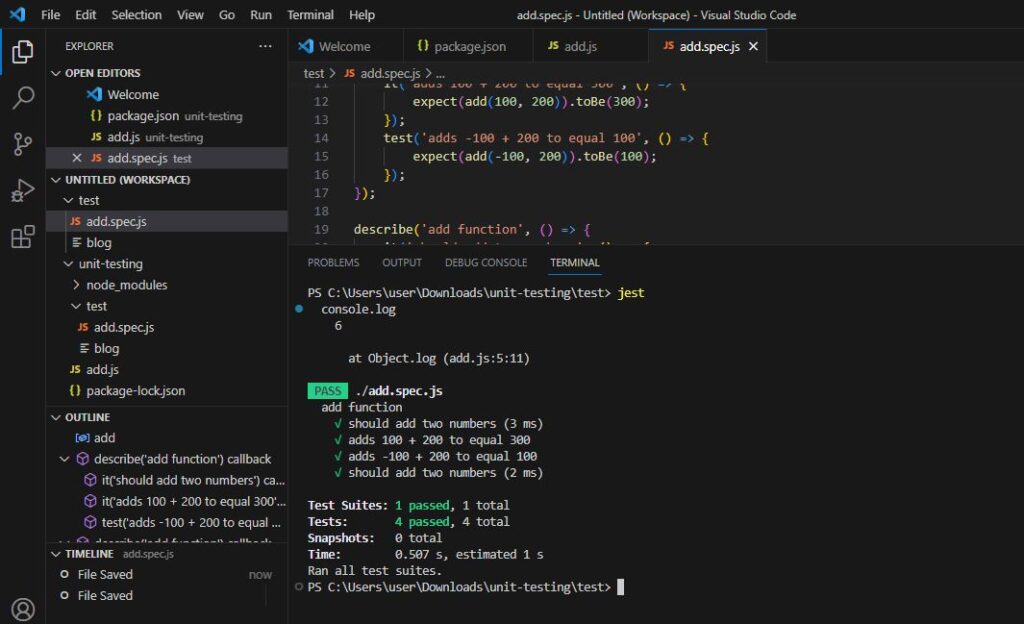
Conclusion
So, these are the everything relates to the unit testing for NodeJS using Jest Framework. Finally, we can say that though there are several JS test frameworks available, these are the reasons why one can choose Jest as a preference:
- Jest is easy to install and set up, and it comes with built-in support for popular tools like Babel and TypeScript.
- Jest is designed to be fast and reliable.
- Jest has enough support with great documentation that makes it easy to start, learn, and proceed.
- It also provides great community support.
- Overall, Jest is a powerful and versatile testing framework.
Reference
Authors
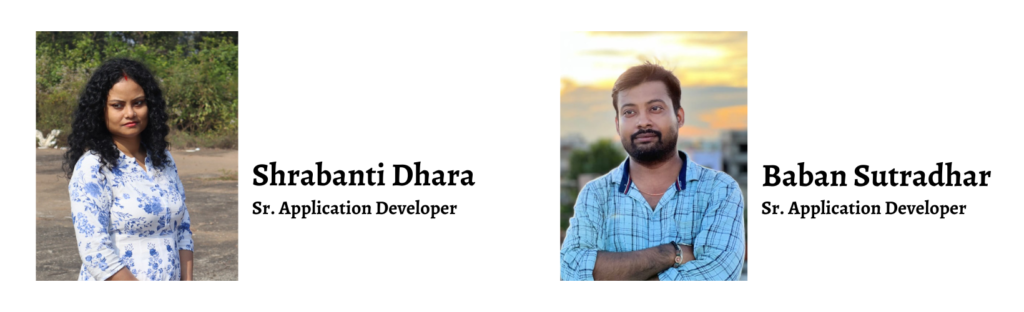