Table of Contents
ToggleWhat is Redux Toolkit?
To Understand What is Redux toolkit, We need to understand, what is Redux?
Redux is an open source Javascript library which is used for managing the state of applications,especially in complex web applications and user interfaces.
The key components that enable the centralized approach to state management in Redux are:
Store: The store is the single source of truth and represents the entire state of the application. It holds the state data and provides methods to update the state. Components can access the store to retrieve data and subscribe to changes in the state.
State: The state is a plain JavaScript object that holds the data representing the application’s current state. The state is read-only, and any changes to it are made by dispatching actions.
Actions: Actions are just plain JavaScript objects that represent what happened in the application. They contain a type property (a string constant) that indicates the type of action being performed and additional data if required. Actions are dispatched to the store to trigger state changes.
Reducers: Reducers are pure functions responsible for handling actions and producing the next state of the application. They take the current state and an action as input and return a new state based on the action type. Reducers must be pure functions, meaning they should not modify the original state but instead produce a new state object.
Dispatch: Dispatch is a method provided by the store that allows components to send actions to the store. When an action is dispatched, it is passed to the reducers, which update the state accordingly.
Subscribers: Components can subscribe to the store to receive updates whenever the state changes. This enables components to stay in sync with the centralized state and update their rendering based on the latest data.
How to install Redux Toolkit?
To install Redux Toolkit, You can use npm or yarn, which are popular package managers for Javascript. Here’s how you can install Redux Toolkit using npm or yarn:
npm install @reduxjs/toolkit
or
yarn add @reduxjs/toolkit
How to create a slice
After you have installed the needed dependencies, create a new “slice” using the createSlice function. A slice is a portion of the Redux store that is responsible for managing a specific piece of state.
import { createSlice } from "@reduxjs/toolkit";
const initialState = {
tasks: [],
};
const todoSlice = createSlice({
name: "todo",
initialState,
reducers: {
addTodo: (state, action) => {
state.tasks.push({ id: Date.now(), text: action.payload });
},
deleteTodo: (state, action) => {
state.tasks = state.tasks.filter((task) => task.id !== action.payload);
},
},
});
export const { addTodo, deleteTodo } = todoSlice.actions;
export default todoSlice.reducer;
How to set up Redux Store?
The most basic way to create a store is to use the configureStore() function, which automatically generates a root reducer for you by combining all the reducers defined in your application.
import { configureStore } from "@reduxjs/toolkit";
import todoReducer from "./features/todo/todoSlice";
const store = configureStore({
reducer: {
todo: todoReducer,
},
});
export default store;
Conclusion
The Redux Toolkit aims to make Redux development more efficient , maintainable, and enjoyable by providing a set of best practices and utilities. It’s important to note that while the Redux toolkit can simplify many aspects of Redux, a basic understanding of Redux concepts is still necessary. It provides a predictable and centralized way to manage the state of an application, making it easier to maintain, test, and reason about state changes.
Authors
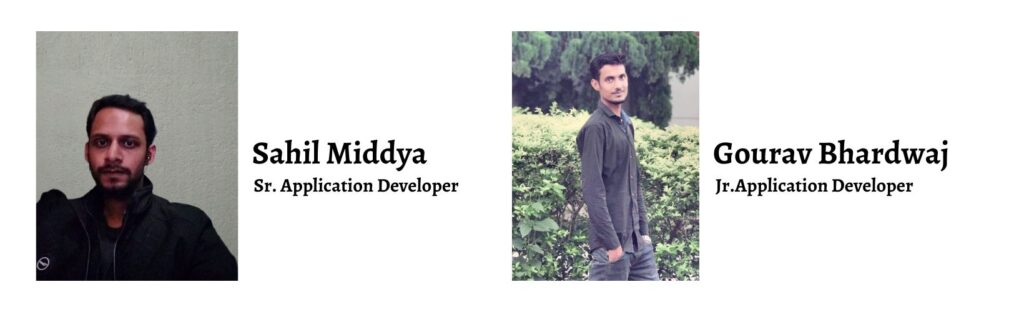